Ace Your Java Interview: Top 500+ Questions to Know
Q1. Why Java is a platform independent language?
Java is a platform-independent language because it runs on a virtual machine called the Java Virtual Machine (JVM). This means that Java code can be written once and run on any platform or operating system that has a JVM installed.
The JVM acts as an intermediary between the Java code and the underlying operating system, allowing it to execute the code in a way that is consistent across different environments. This makes Java an ideal language for developing cross-platform applications that can be deployed on multiple devices and operating systems.
Java applications are called WORA(Write Once Run Anywhere).
Q2. What is Java?
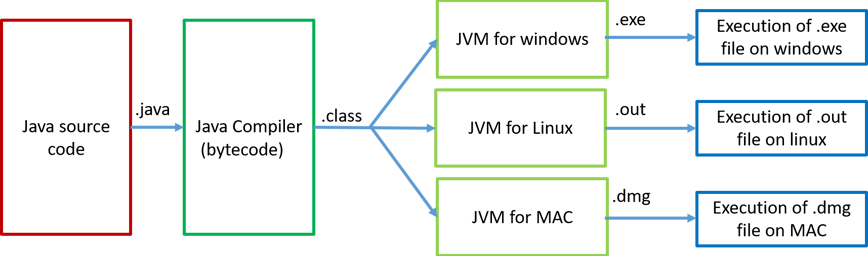
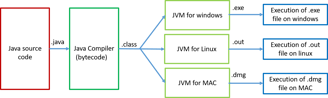
Java is a high-level, object-oriented programming language that is widely used for developing applications and software for various platforms. It was created in the mid-1990s by James Gosling and his team at Sun Microsystems.
Java code is platform-independent, meaning it can be run on any device or operating system that has a Java Virtual Machine (JVM) installed.
Java is used for developing a wide range of applications, including web applications, mobile applications, and enterprise software. Its versatility and cross-platform compatibility have made it one of the most popular programming languages in the world.
Q3. What are the main features of Java?
i) Object Oriented: Java is an object-oriented language where everything is done keeping objects (data) in mind.
ii) Simple: Java is very easy to learn and follow. It's syntax is very easy. Any programmer who has some basic knowledge of object-oriented languages like C++ can easily follow Java.
iii) Platform Independent: Java is a write-once, run-everywhere(WORA) language. That means Java programs written on one platform can be run on any other platform without much difficulty.
iv) Secured: Java is a highly secured language through which you can develop virus-free and highly secured applications.
v) Robust: Java is robust because of automatic garbage collection, better exception, and error handling mechanism, no explicit use of pointers, and a better memory management system.
vi) Portable: Java is portable because you can run Java bytecode on any hardware which has compliant JVM which converts bytecode according to that particular hardware.
vii) Multithreaded: Java supports multithreaded programming where multiple threads execute their task simultaneously.
viii) Distributed: Java is distributed because you can develop distributed large applications using Java concepts like RMI and EJB.
ix) Dynamic: Java is a dynamic language because it supports the loading of classes on demand.
x) Extensible: You can develop new classes using existing interfaces, you can declare new methods to existing classes or you can develop new sub-classes to existing classes. That is all because of the extensible nature of Java.
xi) Functional Style Programming: With the introduction of lambda expressions, functional interfaces, and Stream API in Java 8, you can also write a functional style of programming in Java.
Q4. Write a program to print “Hello World” in Java?
Q5. Why Java is not a pure Object Oriented programming language?
There are six qualities to be satisfied for a programming language to be purely Object Oriented:
a. Encapsulation/Data Hiding
b. Inheritance
c. Polymorphism
d. Abstraction
e. All predefined types are objects
f. All user-defined types are objects
Java supports primitive data types - byte, boolean, char, short, int, float, long, and double and hence it is not an object-oriented language. These primitive data types can be used without the use of any object.
Q6. What is the difference between JDK, JRE and JVM?
JDK, JRE, and JVM are three essential components of the Java programming language.
i) JDK: JDK stands for Java Development Kit, which includes tools for developing, debugging, and documenting Java programs. It also includes a compiler to convert Java code into Bytecode.
ii) JRE: JRE stands for Java Runtime Environment, which is required for running Java applications on a machine. It includes the JVM and class libraries.
iii) JVM: JVM stands for Java Virtual Machine, a virtual machine that executes the Bytecode generated by the Java compiler. It provides a layer of abstraction between the Java program and the operating system, allowing the program to run on any machine that has a JVM installed. Understanding the difference between these three components is crucial for Java developers to develop and run Java applications smoothly.
Q7. What is a final variable?
The final variable is a variable constant that cannot be changed once initialized.
Q8. What are the differences between C++ and Java?
Q9. What is JVM?
JVM stands for Java Virtual Machine. It is a virtual machine that runs Java bytecode and interprets it into machine language. In simple terms, JVM acts as an intermediary between the compiled Java code and the hardware on which it runs. It provides a platform-independent environment for executing Java applications on any device or operating system.
Q10. What is the OOPS concept?
Java OOPS concepts refer to the fundamental principles of Object-Oriented Programming in the Java programming language. These concepts are crucial in understanding and creating robust and efficient Java applications.
The four main pillars of Java OOPS concepts are :
Encapsulation: Encapsulation ensures that the implementation details of a class are hidden, allowing for better data security and code maintainability.
Inheritance: Inheritance enables the creation of new classes by inheriting the properties and behaviors of existing classes, promoting code reusability.
Polymorphism: Polymorphism allows objects to take on multiple forms, enabling flexibility and extensibility in the code.
Abstraction: Abstraction focuses on representing real-world entities as classes and objects, simplifying complex systems, and improving code readability.
By mastering these Java OOPS concepts, developers can design and develop scalable, modular, and robust Java applications.
Q11. What are the types of Inheritance supported in Java?
In Java, there are several types of inheritance that are supported.
The first type is single inheritance, which means that a class can inherit properties and methods from only one parent class.
The second type is multiple inheritance, which is not supported in Java. This means that a class cannot inherit from multiple parent classes. However, Java does support multiple interface inheritance, which allows a class to implement multiple interfaces.
Another type of inheritance in Java is hierarchical inheritance, where a class can have one parent class and multiple child classes.
Lastly, there is hybrid inheritance, which is a combination of single and multiple inheritance. Overall, Java provides different types of inheritance to support various class relationships and facilitate code reusability.
Q12. Why is multiple inheritance not supported in Java?
Multiple inheritance is not supported in Java because it can lead to various complications and conflicts. In Java, a class can only inherit from one superclass, which helps maintain simplicity and avoids ambiguity in the code. Unlike other programming languages like C++, Java emphasizes the concept of single inheritance to avoid the diamond problem, where conflicts arise when a class inherits from two classes that share a common superclass. For example, consider a scenario where Class A and Class B have a method with the same name display(), and Class C extends Class A and Class B then it becomes unclear which method should be called when invoking it from Class C. This ambiguity can cause confusion and make the code difficult to maintain. See below example :
Q13. What is the difference between abstract class and interface?
An abstract class and an interface are both used in object-oriented programming to define common attributes and behaviors that can be inherited by other classes. However, there are some key differences between them.
An abstract class can have both abstract and non-abstract methods, while an interface can only have abstract methods. In other words, an abstract class can provide a default implementation for some of its methods, whereas an interface only declares the method signatures.
Another difference is that a class can only inherit from one abstract class, but it can implement multiple interfaces. This allows for more flexibility in terms of code reuse.
Additionally, an abstract class can have instance variables, constructors, and static methods, but an interface can only have constant variables.
Overall, the choice between using an abstract class or an interface depends on the specific needs of the program and the desired level of abstraction and flexibility.
Q14. Is there any default value for Java local variables?
No, local variables are not initialized by any default values.
Q15. What do you mean by JRE?
JRE stands for Java Runtime Environment. It is a software package that provides the necessary runtime components for executing Java applications. The JRE includes the Java Virtual Machine (JVM), which is responsible for executing the Java bytecode, as well as various libraries and class files that are required for running Java programs.
In simpler terms, the JRE allows you to run Java applications on your computer, without needing to compile the source code into machine code. It is an essential component for anyone who wants to run Java applications or applets on their system.
Q16. What is JIT Compiler in Java?
Just-In-Time (JIT) is a concept in Java that refers to a compiler that transforms Java bytecode into native machine code at runtime. The purpose of JIT is to improve the performance of Java programs by optimizing the execution process.
When a Java program is run, the bytecode is interpreted by the Java Virtual Machine (JVM). However, interpretation can be slower compared to executing native machine code. By using the JIT compiler, the JVM can dynamically identify frequently executed code segments and compile them into efficient machine code, resulting in faster program execution.
Q18. What are the different types of memory areas allocated by JVM?
In Java, JVM allocates memory to different processes, methods, and objects. Some of the memory areas allocated by JVM are:
1. ClassLoader: It is a component of JVM used to load class files.
2. Class (Method) Area: It stores per-class structures such as the runtime constant pool, field and method data, and the code for methods.
3. Heap: Heap is created as a runtime and it contains the runtime data area in which objects are allocated.
4. Stack: Stack stores local variables and partial results at runtime. It also helps in method invocation and return value. Each thread creates a private JVM stack at the time of thread creation.
5. Program Counter Register: This memory area contains the address of the Java virtual machine instruction that is currently being executed.
6. Native Method Stack: This area is reserved for all the native methods used in the application.
Q19. What is a JIT compiler?
Just In Time compiler also known as JIT compiler is used for performance improvement in Java. It is enabled by default. It is a compilation done at execution time rather than earlier. Java has popularized the use of the JIT compiler by including it in JVM.
Q17. What are the different types of variables in Java?
In Java, there are three types of variables and they are:
Static Variables
Local Variables
Instance Variables
Static Variables: A variable that is declared with the static keyword in a class is called a static variable. A static variable cannot be a local variable, and the memory is allocated only once for these variables.
Local Variables: A variable that is declared inside the method body within the class is called a local variable. To access a local variable it must be initialized.
Instance Variables: The variable declared inside the class but outside the body of the method is called the instance variable. It's value is instance-specific and cannot be shared among others.
Q20.How Java platform is different from other platforms?
Java is a platform-independent language. Java compiler converts Java code into byte code that can be interpreted by JVM. There are JVM written for almost all the popular platforms in the world. Java byte code can run on any supported platform in the same way. Where as other languages require libraries compiled for a specific platform to run.
Q21.Why do people say that Java is a 'write once and run anywhere' language?
You can write Java code on Windows and compile it on the Windows platform. The class and jar files that you get from the Windows platform can run as it is on a Unix environment. So it is a truly platform-independent language. Behind all this portability is Java byte code. Byte code generated by the Java compiler can be interpreted by any JVM. So it becomes much easier to write programs in Java and expect those to run on any platform. Java compiler javac compiles Java code and JVM Java runs that code.
Q22.Can we write main method as public void static instead of public static void?
No, you cannot write it like this. Any method has to first specify the modifiers and then the return value. The order of modifiers can change. We can write static public void main() instead of public static void main().
26. Java what is the default value of an object reference defined as an instance variable in an Object?
All the instance variable object references in Java are null.
27.Why do we need a constructor in Java?
Java is an object-oriented language, in which we create and use objects. A constructor is a piece of code similar to a method. It is used to create an object and set the initial state of the object. A constructor is a special function that has the same name as class name. Without a constructor, there is no other way to create an object. By default, Java provides a default constructor for every object. If we overload a constructor then we have to implement default constructor.
28.Why do we need a default constructor in Java classes?
A Default constructor is the no-argument constructor that is automatically generated by Java if no other constructor is defined. Java specification says that it will provide a default constructor if there is no overloaded constructor in a class. But it does not say anything about the scenario in which we write an overloaded constructor in a class. We need at least one constructor to create an object, that’s why Java provides a default constructor. When we have an overloaded constructor, Java assumes that we want some custom treatment in our code. Due to which it does not provide a default constructor. But it needs a default constructor as per the specification. So it gives an error.
29.What is the value returned by Constructor in Java?
When we call a constructor in Java, it returns the object created by it. That is how we create new objects in Java.
30.Can we inherit a Constructor?
No, Java does not support the inheritance of constructor.
31. Why constructors cannot be final, static, or abstract in Java?
If we set a method as final it means we do not want any class to override it. However, the constructor (as per Java Language Specification) cannot be overridden. So there is no use of marking it final. If we set a method as abstract it means that it has no body and it should be implemented in a child class. But the constructor is called implicitly when the new keyword is used. Therefore it needs a body. If we set a method as static it means that it belongs to the class, but not a particular object. The constructor is always called to initialize an object. Therefore, there is no use of marking the constructor static.
32.What is the purpose of ‘this’ keyword in java?
In Java, ‘this’ keyword refers to the current instance of the object. It is useful for differentiating between instance variables and local variables. It can be used to call constructors. Or it can be used to refer to the instance. In the case of method overriding, this is used for falling the method of the current class.
33. Explain the concept of Inheritance.
Inheritance is an important concept in Object Oriented Programming. Some objects share certain characteristics and behaviors. By using Inheritance, we can put the common behavior and characteristics in a base class which is also known as super class. Then all the objects with common behavior inherit from this base class. It is also represented by IS-A relationship. Inheritance promotes code reuse, method overriding and polymorphism.
34. Which class in Java is superclass of every other class?
Java is an object-oriented programming language. In Java, the Object class is the superclass of every other class.
35. Why Java does not support multiple inheritance?
Multiple Inheritance means that a class can inherit behavior from two or more parent classes. The issue with Multiple Inheritance is that both the parent classes may have different implementations for the same method. So they have different ways of doing the same thing. Now which implementation should the child class choose? This leads to ambiguity in Multiple Inheritance. This is the main reason for Java does not support Multiple Inheritance in implementation.
Let's say you have a class TV and another class AtomBomb. Both have method switchOn() but only TV has switchOff() method. If your class inherits from both these classes then you have an issue that you can switchOn() both parents, but switchOff will only switchOff() TV. But you can implement multiple interfaces in Java.
36. In OOPS, what is meant by composition?
Composition is also known as “has-a” relationship. In composition, “has-a” relation relates two classes. E.g. Class Car has a steering wheel. If a class holds the instance of another class, then it is called composition.
37. How aggregation and composition are different concepts?
In OOPS, Aggregation and Composition are the types of association relations. A composition is a strong relationship. If the composite object is destroyed, then all its parts are destroyed. E.g. A Car has a Steering Wheel. If the Car object is destroyed, then there is no meaning of the Steering Wheel. In Aggregation, the relationship is weaker than Composition. E.g. A Library has students. If a Library is destroyed, Students still exist. So Library and Student are related by Aggregation. A Library has Books. If the Library is destroyed, the Books are also destroyed. Books of a Library cannot exist without the Library. So Book and Library are related by Composition.
38. Why there are no pointers in Java?
In Java there are references instead of pointers. These references point to objects in memory. But there is no direct access to these memory locations. JVM is free to move the objects within VM memory. The absence of pointers helps Java in managing memory and garbage collection effectively. Also it provides developers with convenience of not getting worried about memory allocation and deallocation.
39. If there are no pointers in Java, then why do we get NullPointerException?
In Java, the pointer equivalent is Object reference. When we use a . it points to object reference. So JVM uses pointers but programmers only see object references. In case an object reference points to null object, and we try to access a method or member variable on it, then we get NullPointerException.
40. What is the purpose of ‘super’ keyword in java?
‘super’ keyword is used in the methods or constructor of a child class. It refers to immediate parent class of an object. By using ‘super’ we can call a method of parent class from the method of a child class. We can also call the constructor of a parent class from the constructor of a child class by using ‘super’ keyword.
41. Is it possible to use this() and super() both in same constructor?
No, Java does not allow using both super() and this() in the same constructor. As per Java specification, super() or this() must be the first statement in a constructor.
42.What is the meaning of object cloning in Java?
Object.clone() method is used for creating an exact copy of the object in Java. It acts like a copy constructor. It creates and returns a copy of the object, with the same class and with all the fields having the same values as of the original object. One disadvantage of cloning is that the return type is an Object. It has to be explicitly cast to the actual type.
Q23.In Java, if we do not specify any value for local variables, then what will be the default value of the local variables?
Java does not initialize local variables with any default value. So these variables will be just null by default
Q24.What are the main principles of Object Oriented Programming?
The Main principles of Object Oriented Programming (OOPS) are:
1. Abstraction
2. Encapsulation
3. Inheritance
4. Polymorphism
Q25.What is the difference between Object Oriented Programming language and Object-based Programming language?
Object Oriented Programming languages like Java and C++ follow concepts of OOPS like- Encapsulation, Abstraction, Polymorphism, Inheritance, etc. Object Based Programming languages follow some features of OOPS but they do not provide support for Polymorphism and Inheritance. Egg. JavaScript, VBScript, etc. Object-based Programming languages provide support for Objects and you can build objects from the constructor. These languages also support Encapsulation. These are also known as Prototype-oriented languages
43. What is the difference between wait(), sleep() and join() method?
The difference between the wait(), sleep(), and join() methods lies in their functionality and purpose.
wait() : This method is used for thread synchronization, allowing a thread to pause and wait for a specific condition to be met before proceeding.
sleep() : This method is utilized to introduce a delay or pause in the execution of a thread for a specified amount of time. This aids in managing thread timing and coordination.
join() : This method is employed to ensure that a thread completes its execution before the main thread terminates. It is useful when we want to wait for a thread to finish its task before moving forward in the program.
44. What is the difference between throw and throws keyword?
The throw keyword is used to manually throw an exception within a block of code. It is typically followed by an instance of the Exception class or one of its subclasses.
On the other hand, the throws keyword is used to declare that a method may throw one or more types of exceptions. It is followed by the names of the exceptions the method might throw, separated by commas. This allows the caller of the method to be aware of the potential exceptions that can be thrown and handle them accordingly.
In summary, throw is used to actually throw an exception, while throws is used to declare that a method might throw an exception.
45. In how many ways we can create a thread in Java?
In Java, there are multiple ways to create a thread.
One way is by extending the Thread class and overriding its run() method. This allows you to define the specific actions that the thread should perform.
Another way is by implementing the Runnable interface and providing the implementation for the run() method. This approach is useful when you want to separate the thread logic from the class hierarchy.
Additionally, you can use the Executor framework, which provides a higher-level abstraction for managing threads. This framework allows you to submit tasks to a thread pool, which then assigns these tasks to available threads. The Executor framework provides greater control and flexibility in managing thread execution.
Overall, these different approaches offer various options for creating threads in Java, catering to different requirements and scenarios.
46. What is Functional Interface?
A functional interface is a type of interface in Java programming language that has only one abstract method. It is also known as a Single Abstract Method (SAM) type. Functional interfaces are used to implement lambda expressions and functional programming concepts in Java. The main purpose of a functional interface is to provide a blueprint for creating functional objects. These objects can be used as parameters or return types in lambda expressions and method references. The use of functional interfaces enhances code readability and enables the implementation of functional programming features in Java. They play a significant role in the development of concise and efficient code.
47. What is the difference between Sequential & Parallel Stream?
Sequential stream and parallel stream are two different modes of processing data in Java streams.
In a sequential stream, the elements are processed one after another in a single thread, meaning that each element is processed only after the previous one has been fully processed. This sequential processing ensures that the order of the elements is maintained.
On the other hand, a parallel stream splits the input into multiple substreams and processes them concurrently in separate threads. This enables the processing of multiple elements simultaneously, potentially improving performance for computationally intensive tasks. However, it is important to note that parallel processing may not guarantee the same order of elements as in the input stream.
Therefore, depending on the specific requirements of the task, choosing between sequential and parallel streams is crucial for achieving the desired outcome efficiently.
48. What is the contract between equals() and hashCode() method?
The contract between the equals() and hashCode() methods is an essential aspect of the Java programming language.
This contract ensures that if two objects are considered equal based on the equals() method (eg. obj1.equals(obj2)), their hash codes should also be equal according to the hashCode() method.
In other words, if two objects have the same hash code, it indicates that they are equal. However, it is important to note that the reverse is not true - two objects with the same hash code are not necessarily equal. The hashCode() method returns an integer value, while equals() method compares objects for equality. By adhering to this contract, developers can maintain consistency and reliability in their code, allowing for efficient usage of data structures such as hash tables and hash sets.